Lab 8: The table method
Due to Fall Break, we will skip this lab.
What do we have, according to the template?
What do we want, according to the examples?
How do we turn what we have into what we want?
The Beginning Student Tables tool is available online for your use.
Exercise 1. (from The table method) Use the table method to design a function ctof that converts a temperature in Celsius to a temperature in Fahrenheit.
Exercise 2. (from The table method) Use the table method to design a function combine-digits that combines two digits (each an integer between 0 and 9) into an integer between 0 and 99. The first given digit should become the first digit of the output (in other words, tens). The second given digit should become the second digit of the output (in other words, ones).
; A Year is a non-negative integer ; A Month is one of: ; - "January" ; - "February" ; - "March" ; - "April" ; - "May" ; - "June" ; - "July" ; - "August" ; - "September" ; - "October" ; - "November" ; - "December" ; A Day is an integer at least 1 but at most 31 ; year-month-day->days : Year Month Day -> Number ; returns the number of days elapsed since January 1, 0 ; given: 0 "January" 1 expect: 0 ; given: 2017 "August" 28 expect: 736444
; month->days-in-year : Month -> Number ; returns the days elapsed in the year before the given month ; given: "January" expect: 0 ; given: "September" expect: 243
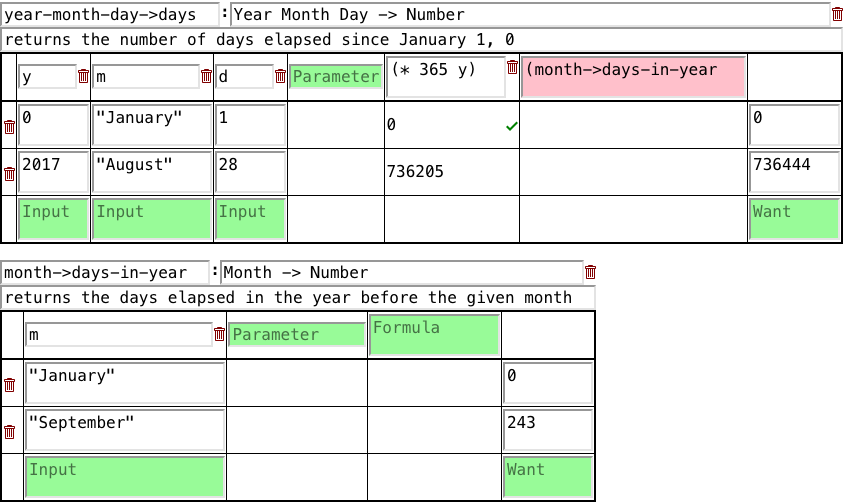
When the input is 0, the yellow text in the output should have size 200.
When the input is 99, the yellow text in the output should have size 2.
When the input is 100 or greater, the text should disappear.
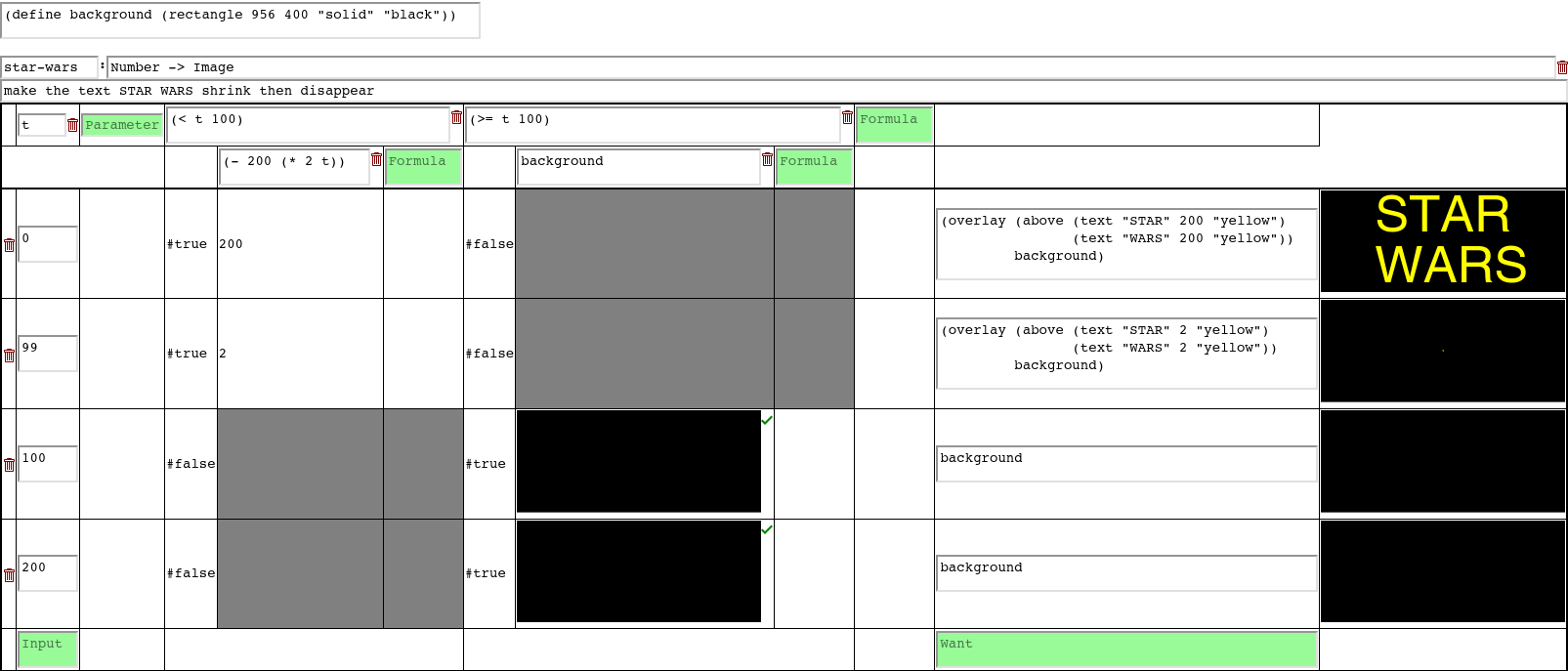
Exercise 5. (from Problem set 5: Enumerations and structures) Use the table method to design a function called format-address which, given an address, produces a string that you might write on a letter to that address. In other words, this function should consume an address data structure and produce a formatted string. The function number->string may be useful.
To start the table, add rows for a variety of input Addresses, and add columns for all the ingredients supplied by the template for processing a Address, like this:
In the Beginning
Student Tables tool, put your data definition for Address and
structure definition for address in the “Definitions Area” at the
top.
Exercise 6. (from Problem set 6: Unions and recursion) Use the table method to design a function salary that accepts a Job and returns its salary, which is the initial salary of the entry plus all the raises of the promotions.
To start the table, add rows for a variety of input Jobs, and add columns for all the ingredients supplied by the template for processing a Job, like this:
In the Beginning
Student Tables tool, put your data definition for Job and
structure definitions for entry and promotion in the
“Definitions Area” at the top.
Exercise 7. (from Problem set 6: Unions and recursion) Use the table method to design a function weight that takes a Mobile as input and computes its total weight.
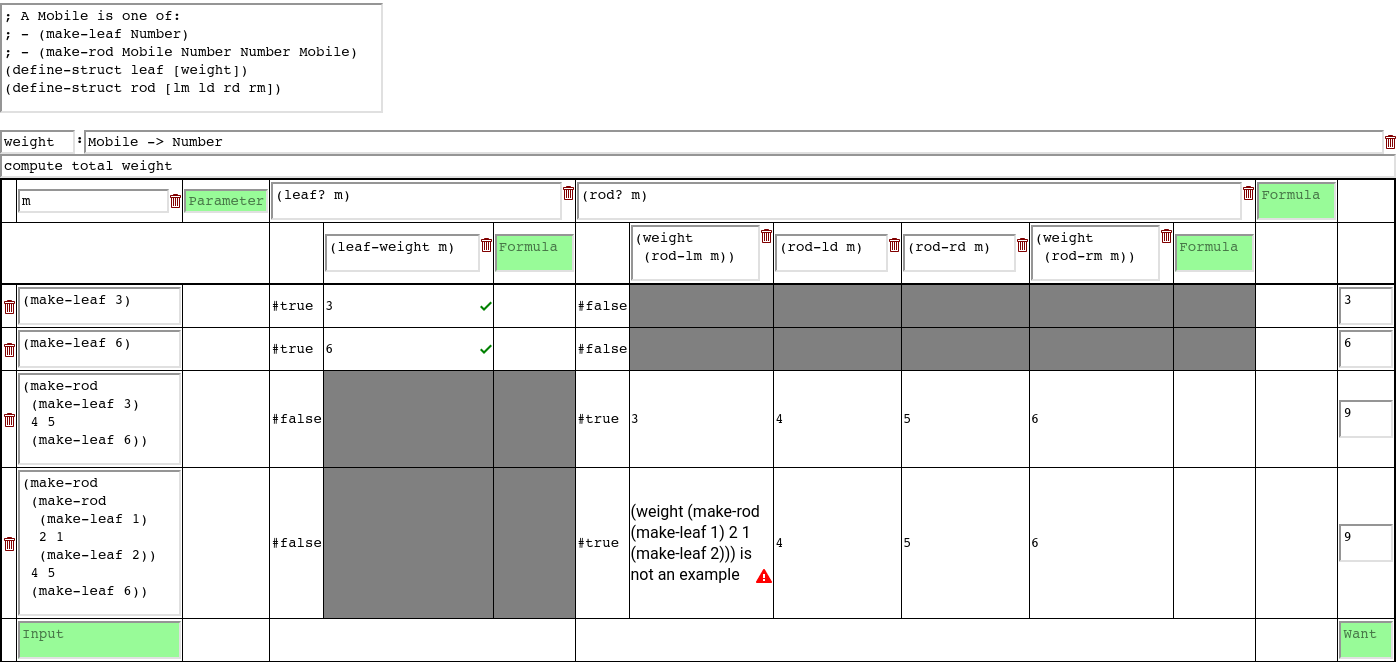
; A Mobile is one of: ; - (make-leaf Number) ; - (make-rod Mobile Number Number Mobile) (define-struct leaf [weight]) (define-struct rod [lm ld rd rm])
Exercise 8. Use the table method to design a function sum that takes a ListOfNumbers as input and computes its sum.
To start the table, add rows for a variety of input
ListsOfNumbers, and add columns for all the ingredients
supplied by the template for processing a ListOfNumbers, like
this:
Exercise 9. Use the table method to design a function add-10-to-all that takes a ListOfNumbers as input and adds 10 to every number in it to produce a new ListOfNumbers.
To start the table, add rows for a variety of input
ListsOfNumbers, and add columns for all the ingredients
supplied by the template for processing a ListOfNumbers, like
this:
Exercise 10. (from Problem set 7: Lists) Use the table method to design a function remove-<=100 that takes a ListOfNumbers as input and removes every number less than or equal to 100 to produce a new ListOfNumbers.
To start the table, add rows for a variety of input
ListsOfNumbers, and add columns for all the ingredients
supplied by the template for processing a ListOfNumbers, like
this:
Exercise 11. Use the table method to design a function remove-first-<=100 that takes a ListOfNumbers as input and removes just the first number less than or equal to 100, if any, to produce a new ListOfNumbers.
To start the table, add rows for a variety of input
ListsOfNumbers, and add columns for all the ingredients
supplied by the template for processing a ListOfNumbers, like
this: